In the vast and often bewildering world of REST APIs, where each endpoint is a gateway to endless possibilities, annotations stand as the unsung heroes, guiding developers through the intricate web of requests and responses. Picture yourself as an explorer, navigating through an ancient, dense jungle. Annotations are your trusty map and compass, providing direction and clarity on your journey. From small teams bewildered by their old codebase to big companies looking to streamline their API documentation, understanding annotations is a step towards enlightenment. Let's embark on this adventure together, exploring the realm of annotations in REST API, where every line of code tells a story, and every annotation is a signpost pointing the way.
What are Annotations in REST API?
Definition and Purpose of Annotations in REST API
Annotations in REST API are like the secret runes that ancient magicians used to invoke their spells. In the practical world of software development, these "runes" are metadata that provide information about how API methods should be used. They help in defining the route, the expected request type, and the response format, turning a complex maze of code into a well-marked trail. By annotating your API, you're essentially embedding instructions directly into your code, making the API self-documenting to some extent and significantly easing the burden on developers to remember the specifics of each endpoint.
Key Takeaway: Annotations act as embedded instructions that guide the usage and behavior of REST API methods, simplifying development and integration.
How Annotations Enhance RESTful Services
Imagine crafting a web service that's as easy to read as your favorite novel, where each chapter seamlessly leads to the next. This is the magic that annotations bring to RESTful services. They enhance clarity, reduce errors, and streamline the development process by explicitly defining the HTTP methods (GET, POST, DELETE, PUT), the paths, and the parameters expected. Annotations ensure that the API behaves predictably for both the developers who build it and the clients who use it, much like a well-drawn map ensures that travelers reach their destination without getting lost.
Key Takeaway: By defining HTTP methods, paths, and parameters, annotations make RESTful services more predictable and easier to use and integrate.
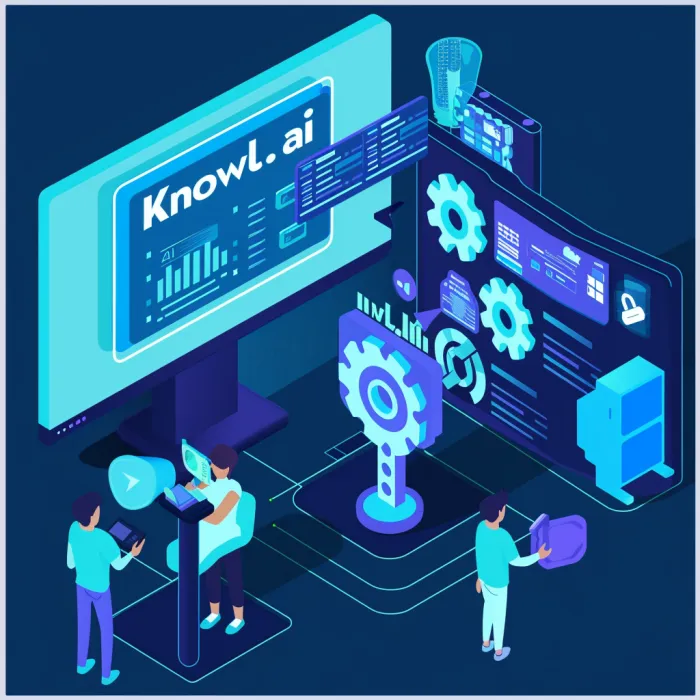
Commonly Used Annotations in REST API
In the toolkit of a REST API developer, certain annotations are as essential as a compass to a sailor. The most commonly used annotations include @GET, @POST, @PUT, and @DELETE, which correspond to the HTTP methods and define what action the API should perform. Annotations like @PathParam and @QueryParam help in specifying how to pass parameters within the URL. For those navigating the seas of Java and frameworks like Spring Boot or JAX-RS, these annotations are the guiding stars, enabling the creation of powerful, efficient RESTful web services.
Key Takeaway: Essential annotations like @GET, @POST, @PUT, @DELETE, @PathParam, and @QueryParam are the building blocks for creating functional and efficient REST APIs.
How to Use Annotations in REST API?
Implementing Annotations in Java for RESTful Services
Sailing the Java sea, where the waters are deep with tradition and rich in history, RESTful services stand as lighthouses, guiding the way for web services. Implementing annotations in Java is akin to charting your course with precision and care. Annotations like @RestController and @RequestMapping at the class level serve as your declaration of intent, marking your Java class as a REST resource ready to serve the digital populace. On the method level, annotations such as @GetMapping or @PostMapping define the specific HTTP actions your service can take, ensuring that each request finds its rightful handler.
Key Takeaway: In Java, class and method-level annotations clearly define how your RESTful services are discovered and interacted with, streamlining the development process.
Example of Annotating a Controller Class in Spring Boot
Imagine now that you're a craftsman, and Spring Boot is your workshop, replete with tools designed to make building web applications a breeze. Annotating a controller class in Spring Boot is like drawing up the blueprint for a magnificent ship, detailing every room, deck, and sail. With @RestController leading the charge, your class is immediately recognized as a REST endpoint. Utilize @RequestMapping("/path") to specify the URI path for all requests handled by the controller. Then, employ @GetMapping, @PostMapping, @PutMapping, and @DeleteMapping to navigate the vast sea of HTTP requests, each annotation steering the request to the appropriate port.
Key Takeaway: Spring Boot annotations provide a structured blueprint for building and navigating RESTful web services, enhancing development efficiency and clarity.
Working with JAX-RS Annotations for Web Services
As we venture into the realm of JAX-RS, the horizon broadens, revealing a land rich with the potential for RESTful web service creation. JAX-RS annotations act as your compass in this world, guiding the request-response cycle with precision. With @Path setting the course at both the class and method levels, and @GET, @POST, @PUT, and @DELETE defining the actions akin to the winds propelling your ship forward, your web service is a vessel well-equipped for the journey. Utilize @PathParam and @QueryParam to fine-tune your navigation, ensuring that each request is met with the right response.
Key Takeaway: JAX-RS annotations are the cartographers of your web service, mapping out the route and actions for efficient and effective REST API development.
Best Practices for Using Annotations in APIs
Optimizing API Development with Annotations
Imagine annotations as your API's DNA—compact, encoded instructions that guide its interaction within the digital ecosystem. To optimize API development, it's crucial to use annotations judiciously. They should clearly define endpoint behaviors, request methods, and expected response types, acting as signposts that lead developers and API consumers alike through a seamless journey. Employing annotations such as @GetMapping or @PostMapping in Spring MVC not only simplifies routing but also enhances readability, making your API a well-documented, navigable entity.
Key Takeaway: Use annotations to clearly define API behaviors and routes, enhancing both developer experience and API usability.
Annotating URLs and Parameters in REST APIs
Navigating a REST API without properly annotated URLs and parameters is like embarking on a treasure hunt without a map. Annotations such as @RequestParam in Spring MVC for parsing query parameters or @PathVariable for embedding values directly in the URL path turn chaotic quests into structured journeys. By specifying method arguments with these annotations, you make your API's intentions transparent, allowing for intuitive interaction and reducing the chance of miscommunication between the client and the server.
Key Takeaway: Precisely annotate URLs and parameters to ensure clear, intuitive paths through your API, making every request a step towards the right treasure.
Handling Media Types Using Annotations
In the diverse landscape of the web, content comes in many forms—JSON, XML, plain text, and more. Just as a skilled chef knows which ingredients to combine for the perfect dish, a savvy developer uses annotations like @Produces and @Consumes to specify the media types an API can produce and consume. This clarity prevents the culinary disaster of serving a JSON response to a client expecting XML, ensuring that the API and its consumers speak the same language.
Key Takeaway: Specify acceptable request and response media types using annotations to prevent misinterpretations and ensure smooth communication between the API and its clients.
Common Challenges with Annotations in REST APIs
Dealing with Annotation Overuse in Java REST API Development
In the realm of Java REST API development, annotations wield the power to simplify and streamline, yet their overuse can ensnare your code in a thicket of complexity. Imagine a library where every book is wrapped and labeled with dozens of tags—finding the book you need becomes a daunting task. Similarly, excessive annotations can obscure the essence of your code, making it difficult to navigate and maintain. To combat this, consider adopting a minimalist approach, using only those annotations that genuinely add value to your API's clarity and functionality. Remember, in the art of coding, sometimes less is indeed more.
Key Takeaway: Use annotations judiciously to avoid cluttering your code, ensuring your API remains navigable and maintainable.
Understanding Annotation Errors and Debugging in RESTful Applications
Embarking on a debugging expedition in the dense jungles of RESTful applications, annotation errors can be elusive beasts, hiding in the underbrush and pouncing when least expected. These errors often stem from misconfiguration or incorrect usage, leading to unexpected behavior or outright failure of your API. To tame these wild errors, arm yourself with a thorough understanding of each annotation's purpose and requirements. Delve into the documentation, experiment in a controlled environment, and don't hesitate to use tools designed to sniff out and highlight annotation missteps.
Key Takeaway: Equip yourself with knowledge and the right tools to effectively identify and correct annotation errors, ensuring the smooth operation of your RESTful applications.
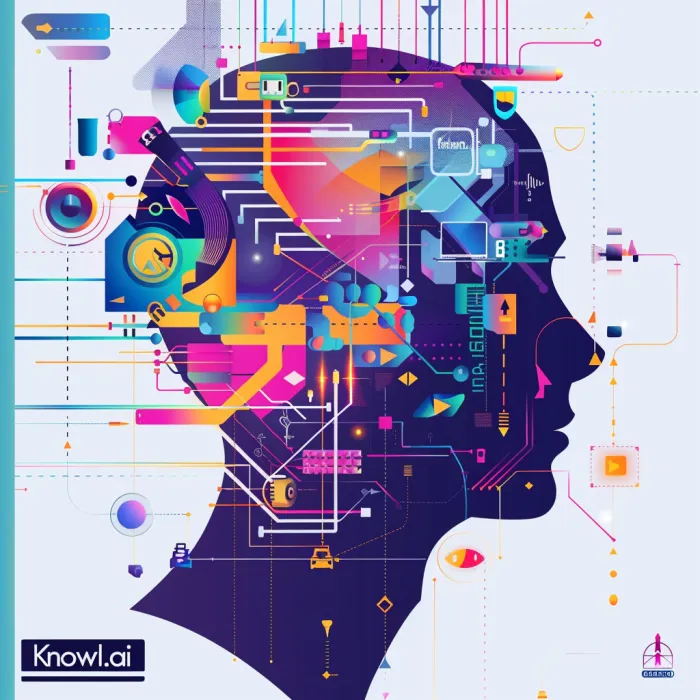
Versioning and Updating Annotations in REST APIs
Imagine your REST API as a living, breathing entity, evolving over time. Just as a snake sheds its skin, your API must shed outdated annotations to embrace new features and improvements. Versioning and updating annotations are akin to navigating through shifting sands—without a clear strategy, you risk getting lost. To maintain your bearings, adopt a versioning scheme that clearly communicates changes to your consumers. Use version numbers in your annotations thoughtfully and consistently, ensuring that each update enhances compatibility rather than causing disruption.
Key Takeaway: Implement a clear and consistent versioning strategy for your annotations to facilitate smooth transitions and maintain compatibility as your API evolves.
Navigating Advanced Waters: Annotations in REST API Development
In the ever-evolving landscape of REST API development, annotations stand as lighthouses guiding developers through the murky waters of code complexity and service optimization. With Knowl.ai as our compass, identifying endpoints and parameters with precision, the journey to mastering advanced annotations becomes a thrilling adventure rather than a daunting task. From the intrepid explorers tackling the wilds of an old codebase to the visionary architects designing the digital infrastructures of tomorrow, annotations in REST API are the runes of power, enabling clarity, efficiency, and innovation. Let's set sail into the advanced realms of annotations, uncovering the secrets that lie within.
Advanced Topics in Annotations and REST API Development
Using PathParam and FormParam Annotations Effectively
In the quest to create intuitive and efficient RESTful applications, the @PathParam and @FormParam annotations emerge as crucial tools. Imagine a scenario where you're navigating through a dense forest, and each path leads to a different destination—a treasure trove of information. The @PathParam annotation allows you to specify dynamic parts of the URI, acting like signposts that guide client requests to the correct resource. On the other hand, @FormParam is akin to discovering hidden compartments within a treasure chest, enabling you to extract data from the form submissions of POST requests effortlessly.
Key Takeaway: Leverage @PathParam for dynamic URI mapping and @FormParam for accessing form submission data, enhancing the flexibility and usability of your RESTful services.
Exploring Class-Level and Method-Level Annotations in Spring Framework
Embarking further into the realm of REST API development, the Spring Framework offers a rich tapestry of class-level and method-level annotations, each serving as a spell to conjure specific behaviors. Class-level annotations, like @RestController, declare the entire class as a RESTful controller, ready to embark on HTTP adventures. Method-level annotations, such as @GetMapping or @PostMapping, specify the HTTP methods to handle, turning each function into a well-defined action point for client requests. Together, these annotations form the backbone of a RESTful application using Spring, orchestrating the flow of requests and responses with precision and grace.
Key Takeaway: Use Spring Framework's class-level and method-level annotations to define and organize your RESTful application's behaviors and endpoints methodically.
Custom Annotations and JAX-RS Extensions for RESTful Web Services
As our journey takes us deeper into uncharted territories, the creation of custom annotations and the exploration of JAX-RS extensions become the tools of legends, allowing developers to tailor their RESTful applications to the unique demands of their quests. Custom annotations in Java can be designed to encapsulate specific behaviors or configurations, offering a way to apply common patterns across multiple endpoints with a single, magical incantation. Meanwhile, JAX-RS extensions provide the flexibility to extend the standard RESTful functionalities, enabling your services to handle different, more complex scenarios with ease and agility.
Key Takeaway: Craft custom annotations for repetitive patterns and utilize JAX-RS extensions to enhance your RESTful web services' capabilities, ensuring your application is both powerful and adaptable.
Fun Fact
Did you know that the concept of annotations in Java was not introduced until Java 5 in 2004? This means that for almost a decade, Java developers navigated the seas of code without the guiding light of annotations, relying on external configuration files to manage behavior that annotations now simplify directly within the code.
FAQs about Annotations in REST API Development
1. What is the primary benefit of using @PathParam in REST APIs?
@PathParam allows for the dynamic inclusion of values in the URI path, making APIs more flexible and capable of handling a wide range of client requests with concise, readable URLs.
2. How do class-level annotations differ from method-level annotations in Spring?
Class-level annotations in Spring, like @RestController, define broad behaviors applicable to the entire class, whereas method-level annotations, such as @GetMapping, specify behaviors for individual methods or actions.
3. Can I create custom annotations for RESTful services, and why would I?
Yes, you can create custom annotations to encapsulate and reuse specific patterns or configurations across your application, enhancing code readability and reducing redundancy.
4. What's the advantage of using JAX-RS extensions in REST API development?
JAX-RS extensions allow developers to extend the standard functionality of their RESTful services, offering more flexibility to meet unique application requirements and handle complex scenarios.
About Knowl.io
Introducing Knowl.io, the revolutionary AI-driven platform designed to transform how API documentation is created and maintained. Say goodbye to the painstaking process of manually updating specifications with each code change—Knowl.io does the heavy lifting for you. With seamless integration into your development workflow, Knowl.io ensures your API documentation is perpetually accurate, reflecting the latest updates in your codebase without the need for manual annotations or explanations.
At the heart of Knowl.io is cutting-edge AI technology that meticulously identifies endpoints, parameters, and behaviors, crafting detailed and up-to-date API documentation with comprehensive explanations. Trust Knowl.io to elevate your documentation process, making it more efficient and reliable than ever. Ensure your developers and stakeholders always have access to the most current and coherent API documentation with Knowl.io, where innovation meets simplicity.